In previous post,we have seen how to develop JAX-WS web service end point and client.In this post,we will see how we can deploy web service end point to application server.
Here we will use tomcat as application server.
1) Open eclipse.
2) Create new web project named "HelloWorldWS"
3) Create new package named "org.arpit.javapostsforlearning.webservice"
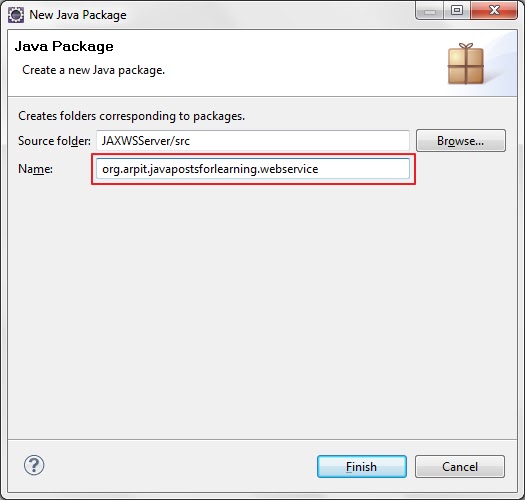
4) Create JAXWSService Interface.
HelloWorld.java
HelloWorldImpl.java
6) Now , you need generate Web Services classes, open your command line, and type :
8) Now we will add sun-jaxws.xml under HelloWorldWS/WebContent/WEB-INF/.It will have endpoint definition.
Web service Tutorial Content:
Part-1:Introduction to web services
Part-2:SOAP web service introduction
Part-3:SOAP web service example in java using eclipse
Part-4:JAX-WS web service eclipse tutorial
Part-5:JAX-WS web service deployment on tomcat
Here we will use tomcat as application server.
1) Open eclipse.
2) Create new web project named "HelloWorldWS"
3) Create new package named "org.arpit.javapostsforlearning.webservice"
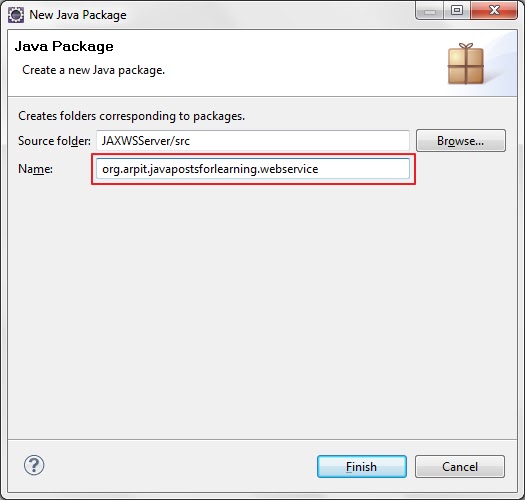
4) Create JAXWSService Interface.
HelloWorld.java
package org.arpit.javapostsforlearning.webservice;5)Create JAXWSService implementation class.
import javax.jws.WebMethod;
import javax.jws.WebService;
@WebService
public interface HelloWorld {
@WebMethod public String helloWorld(String name);
}
HelloWorldImpl.java
package org.arpit.javapostsforlearning.webservice;
import javax.jws.WebService;
@WebService(endpointInterface="org.arpit.javapostsforlearning.webservice.HelloWorld")
public class HelloWorldImpl implements HelloWorld{
public String helloWorld(String name) {
return "Hello world from "+name;
}
}
6) Now , you need generate Web Services classes, open your command line, and type :
cd %project_home%
wsgen -s src -d build/classes -cp build/classes org.arpit.javapostsforlearning.webservice.HelloworldImpl
7)Now you need to create web.xml and put it under /HelloWorldWS/WebContent/WEB-INF/
<?xml version="1.0" encoding="UTF-8"?>we have inserted three things here 1.listner-class 2.servlet 3.servlet-mapping
<web-app
xmlns="http://java.sun.com/xml/ns/j2ee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/j2ee
http://java.sun.com/xml/ns/j2ee/web-app_2_4.xsd"
version="2.4">
<display-name>HellooWorldWS</display-name>
<listener>
<listener-class>
com.sun.xml.ws.transport.http.servlet.WSServletContextListener
</listener-class>
</listener>
<servlet>
<servlet-name>HelloWorldWS</servlet-name>
<servlet-class>
com.sun.xml.ws.transport.http.servlet.WSServlet
</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>HelloWorldWS</servlet-name>
<url-pattern>/HelloWorldWS</url-pattern>
</servlet-mapping>
</web-app>
8) Now we will add sun-jaxws.xml under HelloWorldWS/WebContent/WEB-INF/.It will have endpoint definition.
<?xml version="1.0" encoding="UTF-8"?>
<endpoints xmlns="http://java.sun.com/xml/ns/jax-ws/ri/runtime" version="2.0">
<endpoint
name="HelloWorldWS"
implementation="org.arpit.javapostsforlearning.webservice.HelloWorldImpl"
url-pattern="/HelloWorldWS"/>
</endpoints>
9) We need to download some jaxws jars and put jars under /HelloWorldWS/WebContent/WEB-INF/lib.
you can download attached library from attachment.
10) Now export your project as war and put it under your Tomcat webapps folder.
11) Run tomcat
If You see web service information page then you are done.
Source:Download
No comments:
Post a Comment