The
Package java.util.zip provides classes for reading and writing the standard ZIP and GZIP file formats. This example demonstrate how to open file with JavaFX FileChooser, and compress to .gz file with java.util.zip.GZIPOutputStream.
package javafx_zip;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.logging.Level;
import java.util.logging.Logger;
import java.util.zip.GZIPOutputStream;
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.layout.StackPane;
import javafx.scene.layout.VBox;
import javafx.stage.FileChooser;
import javafx.stage.Stage;
/**
*
* @web http://java-buddy.blogspot.com/
*/
public class JavaFX_Zip extends Application {
File fileSrc;
private static final int bufferSize = 8192;
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("Hello World!");
final Label labelFile = new Label();
Button btn = new Button();
btn.setText("Open FileChooser'");
btn.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
FileChooser fileChooser = new FileChooser();
//Set extension filter
FileChooser.ExtensionFilter extFilter =
new FileChooser.ExtensionFilter("files (*.*)", "*.*");
fileChooser.getExtensionFilters().add(extFilter);
//Show open file dialog
fileSrc = fileChooser.showOpenDialog(null);
String source = fileSrc.getPath();
String targetZipFile = source + ".gz";
try {
//Compress file
GZIPOutputStream gZIPOutputStream;
FileOutputStream fileOutputStream =
new FileOutputStream(targetZipFile);
gZIPOutputStream = new GZIPOutputStream(fileOutputStream);
byte[] buffer = new byte[bufferSize];
try (FileInputStream fileInputStream = new FileInputStream(source)) {
int numberOfByte;
while((numberOfByte = fileInputStream.read(buffer, 0, bufferSize))
!= -1){
gZIPOutputStream.write(buffer, 0, numberOfByte);
}
}
gZIPOutputStream.close();
labelFile.setText("Compressed file saved as: " + targetZipFile);
} catch (IOException ex) {
Logger.getLogger(JavaFX_Zip.class.getName()).log(Level.SEVERE, null, ex);
}
}
});
VBox vBox = new VBox();
vBox.getChildren().addAll(labelFile, btn);
StackPane root = new StackPane();
root.getChildren().add(vBox);
primaryStage.setScene(new Scene(root, 300, 250));
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
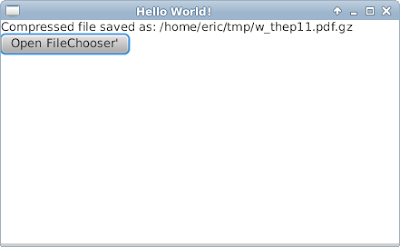 |
Compress file using java.util.zip, with JavaFX interface |
Related:
Decompress file (.gz) using java.util.zip, with JavaFX interface